Today we will handle a case of “Premature Optimization Is the Root of All Evil“.
But this is my blog and I was working with a very big api set, and will only get bigger, so (premature) thinking about memory usage and execution time might be a good idea in the long run.
Before I started this I thought that a for
loop was faster then a foreach
loop. And I usually pick foreach because it’s easier to write and read.
A quick google lands on a stackoverflow question which concludes the opposite. So I started to test a bit.
There is a big difference in my use case here.
I need to remove array items that need to be excluded from the api results. Most examples you will find online are about editing items.
First tests where quite clear, using a foreach
was in most configurations faster than for
. The test array I create has 10000 items and every 3rth item should be excluded:
<?php
$test_array = array();
for ( $i = 0; $i <= 10000; $i ++ ) {
$test_array[] = [
'index' => $i,
'include' => ( $i % 3 === 0 ) ? true : false,
];
}
The traditional foreach loop
The way I have been filtering arrays for years.
Create a empty array and only put in the elements the need to be included.
<?php
$filtered_array = [];
foreach ( $test_array as $item ) {
if ( true === $item['include'] ) {
$filtered_array[] = $item;
}
}
return $filtered_array;
Immediately delete item
Don’t use a between array, just unset the item in the parent array
<?php
foreach ( $test_array as $key => $item ) {
if ( true === $item['include'] ) {
unset( $test_array[ $key ] );
}
}
return $test_array;
Traditional pass by reference
The same as before, but the $item
is passed by reference.
This is the big difference, since we are not editing the $item
we want to remove it from the parent array
<?php
$filtered_array = [];
foreach ( $test_array as &$item ) {
if ( true === $item['include'] ) {
$filtered_array[] = $item;
}
}
return $filtered_array;
Immediately delete pass by reference
Again the same and again passed by reference
<?php
foreach ( $test_array as $key => &$item ) {
if ( true === $item['include'] ) {
unset( $test_array[ $key ] );
}
}
return $test_array;
The for loop
And last the for loop I was wondering about
<?php
$length = count( $test_array );
for ( $i = 0; $i < $length; $i ++ ) {
if ( false === $test_array[ $i ]['include'] ) {
unset( $test_array[ $i ] );
}
}
return $test_array;
the results
I ran each of these loops 5000 times and measured the total time that took.
This was to insure the time between results was big enough to exclude the randomness (at least enough)
The test code I ran
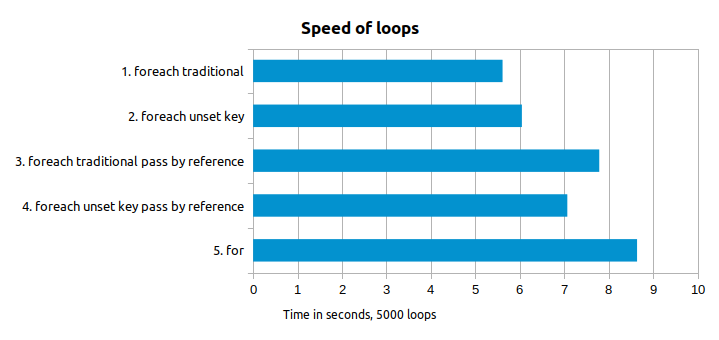
- 5.6122910976414sec Loop: foreach traditional
- 6.0467801094055sec Loop: foreach unset key
- 7.7878839969635sec Loop: foreach traditional pass by reference
- 7.0686309337616sec Loop: foreach unset key pass by reference
- 8.6388339996338sec Loop: for
The traditional foreach I’ve been using for years turned out to be the fastest.
Research hours well spend ?
Bonus edit array item
As said before most examples use editing a array.
So I also ran that scenario. Test code
I upped the loops from 5000 runs to 7500 because the difference was so small.
And still it’s close.
- 15.363855123523sec Loop: foreach traditional
- 10.987272024155sec Loop: foreach foreach edit array directly
- 11.358484983444sec Loop: foreach traditional by reference
- 14.363346099854sec Loop: for
Here the traditional is the slowest. Reference is a lot faster as most articles claim.
But editing the array directly was the fastest.